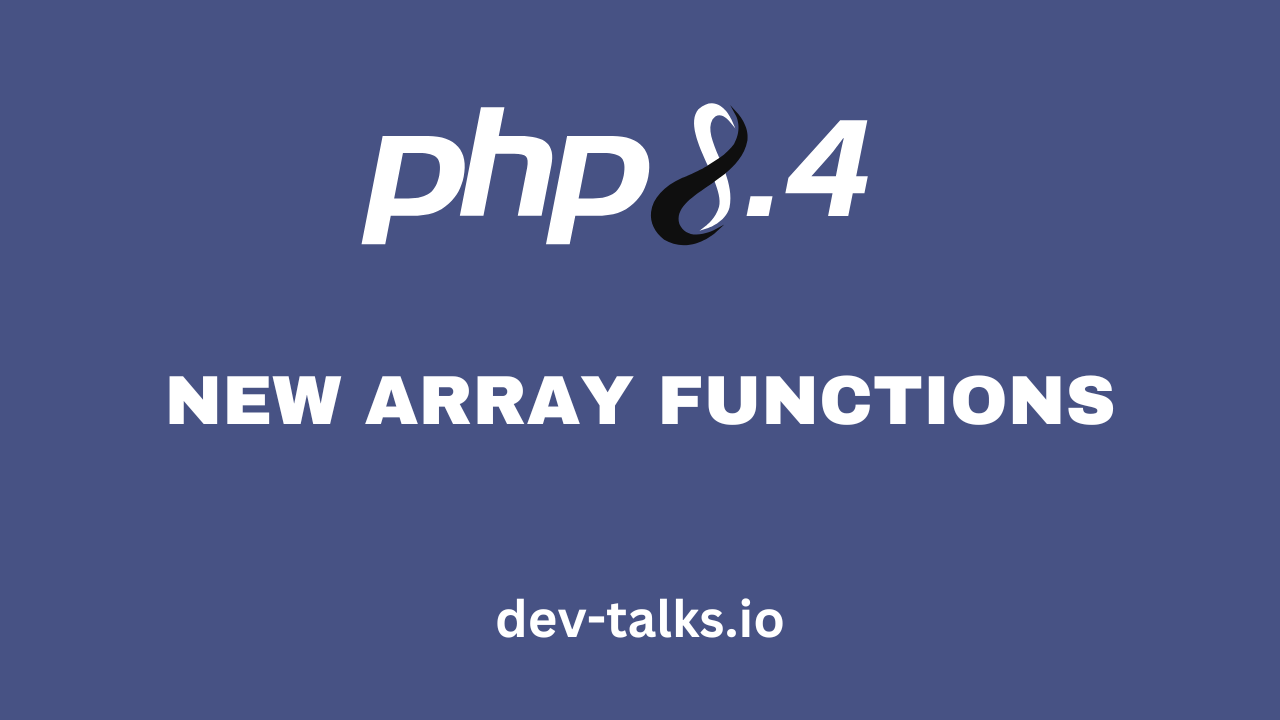
Overview of The New Array Functions in PHP 8.4
Published on Dec 25, 2024
PHP 8.4 introduced four new array functions array_find(), array_find_key(), array_any(), and array_all(). These additions simplify common tasks, reduce boilerplate code, and enhance array handling capabilities.
Let's try to simplify them and find out how we can use them.
1. array_find: Finding the First Match
array_find retrieves the first value in an array that meets a specified condition.
Example: Find the first product costing more than $50.
<php
$products = [20, 40, 60, 80]; $expensiveProduct = array_find($products, fn($price) => $price > 50); echo $expensiveProduct; // Output: 60
2. array_find_key: Locating the First Matching Key
array_find_key gets the first key in an array that satisfies a given condition.
Example: Find the first user older than 18.
<php
$users = ['Ahmed' => 17, 'Ali' => 19, 'Heba' => 15]; $adult = array_find_key($users, fn($age) => $age > 18); echo $adult; // Output: Ali
3. array_any: Checking If Any Element Matches
array_any checks if at least one element in an array meets a specific condition.
Example: Check if a shopping cart has any discounted items:
<?php
$cart = [ ['name' => 'T-shirt', 'discounted' => false], ['name' => 'Jeans', 'discounted' => true], ['name' => 'Hat', 'discounted' => false], ]; $hasDiscountedItems = array_any($cart, fn($item) => $item['discounted']); var_dump($hasDiscountedItems); // Output: bool(true)
4. array_all: Ensuring All Elements Match
array_all verifies if all elements in an array satisfy a condition.
Example: Verify if there are any unread notifications:
<php
$notifications = [ ['id' => 1, 'read' => true], ['id' => 2, 'read' => false], ['id' => 3, 'read' => true], ]; $hasUnreadNotifications = array_any($notifications, fn($notification) => !$notification['read']); var_dump($hasUnreadNotifications); // Output: bool(true)
Finally: Let's read the following text from PHP documentation:
There are currently several functions processing arrays using a callback. However still missing are functions to find a single element matching a condition and the closely related functions of checking whether or not there are elements matching a condition. Implementing these functions in userland is relatively simple, but these functions are often required, leading to the wheel being reinvented over and over. Furthermore these type of functions are implemented in other programming languages like Rust, JavaScript or C++, too. Therefore there is a reason to include these functions as standard with the next PHP version. In addition, the implementation of these functions is quite similar to array_filter and relatively trivial to implement, so the maintenance effort should be low.