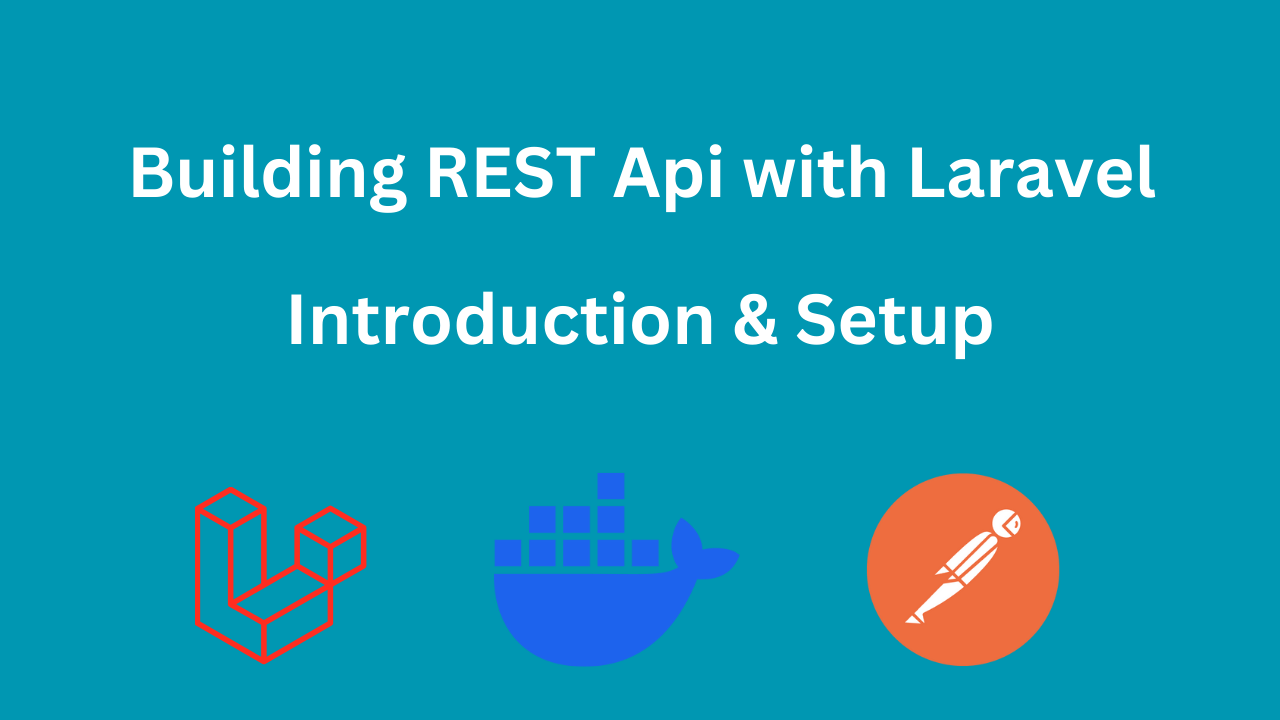
Laravel REST API Part 1: Install with Docker and Sail
Published on Nov 17, 2024
What are we going to build?
We will have a series of articles trying to build a Laravel based RESTful API. I’ll guide you through creating a fully functional API from scratch, covering each step along the way. Whether you’re a beginner or an experienced developer, there’s something valuable for everyone. This series walks you through everything you need to build a robust API step by step.
You can read this series in order or you can jump to a specific topic, It should work either ways, I’ll try to build it that way.
Before we start our setup, let’s first go through some basic concepts.
What’s an API?
API stands for (Application Programming Interface). It’s a way of communication between two applications of or two pieces of software, through some definitions and rules.
What’s a RESTful API?
A RESTful stands for (Representational State Transfer). Simply it’s a type of web API that uses HTTP protocol to enable communication between clients and servers.
Let’s say also it’s the modern way of communication and data exchange of the web services today. In the past people were using a SOAP protocol that was returning XML as response. While in REST we usually return Json.
REST Characteristics
It's Stateless: Meaning that the server doesn’t share information between requests. So for every request you need to send all information to the server to identify that request.
Using HTTP methods:
- GET: Retrieve data (e.g., fetch a user or list of users).
- POST: Create a new resource (e.g., add a new user).
- PUT/PATCH: Update an existing resource (e.g., modify user details).
- DELETE: Remove a resource (e.g., delete a user).
Using also standard HTTP response codes, those are the most used ones:
- 200 OK: Success.
- 201 Created: Resource created successfully.
- 401 Unauthorized: User is not authorized to access this resource.
- 404 Not Found: Resource not found.
- 400 Bad Request: Invalid request.
- 500 Internal Server Error: Server-side error.
Tools Needed for This Project
You need to have PHP, and Composer installed, maybe Docker as well but it’s optional and also we are going to use Postman for testing our Api’s and will Use VSCode with SQLite viewer extension.
We had enough talking, let’s now start building our REST API:
Install Laravel
I’ll suppose you have composer and php and Laravel installed globally
If you do: you need to run this command:
laravel new laravel-rest-api
While the installation process you’ll have some prompts:
- Choose no starter kit
- And for the testing framework, we are going to use PHPUnit
- For database choose SQLite, and you may run the default migration as well this will prompt another question for creating the db file, create it. This way it’ll save some time and setup for us.
You should now done with Laravel installation
Install Sail
If you want to use Docker you need to install Sail which is an awesome Laravel package give you ready setup for docker and docker compose
You can install it by the following command:
php artisan sail:install
Once you run this command you will have a list of services you can choose from, we are going to need Redis and Mailpit for caching and we may do some email stuff so mailpit for testing emails locally.
You’ll now have a docker-compose.yml file created for you. Let’s build our images by the following command:
./vendor/bin/sail up
You can go to your browser now and access our website home page on localhost
And from now on every artisan command could run from inside Sail.
For example:
./vendor/bin/sail artisan make:controller HomeController
This will be same as:
php artisan make:controller HomeController
Whatever you like will be fine.
Congratulations!
You’r setup is complete and we are ready to build our awesome API.